Switch Statement In Dev C++ Example
Sometimes when creating a C++ program, you run into a situation in which you want to compare one thing to a number of other things. Let's say, for example, that you took a character from the user and wanted to compare this to a number of characters to perform different actions. For now, let's just say that you have three commands which operate from the keys: 'h', 'e', and 'q'.
We can use an if-statement to check equality to 'h'
, 'e'
, and 'q'
- remember that we use single quotes because we're dealing with chars, not strings. So let's just write the code in the way we're used to (and let's wrap a while loop around it so it keeps getting a character and acting upon what it was, because that makes our program slightly better and easier to test), using if-statements, like the following:
Hey everyone I'm currently having some trouble in making the switch statement coming back out around to the start of the menu. Instead it proceeds into another main function that I don't want it to. How do I get a switch statement to loop back into a menu. Ask Question Asked 6 years, 1 month ago. C Switch statement loop back to menu. C switch Statements - C switch statement is used when you have multiple possibilities for the if statement. Web Design HTML Tutorials Online HTML, CSS.
This program should be something you're comfortable with creating by this point, however your programmer instincts should also be kicking in and telling you that you shouldn't be repeating so much code here. One of the core principles of object orientated programming is DRY: Don't Repeat Yourself. In this program we're having to repeat a lot of code for the 'else if's including using ch
every time we're doing a comparison.
The solution to this 'problem' is what this tutorial is all about: switch statements. These are essentially just a really nice way to compare one expression to a bunch of different things. They are started via the switch
keyword, and from there comparisons are made using a case:
syntax. It isn't easily described in words, so take a look at the syntax below:
The different case
s essentially act as many if/else-ifs in a chain, and an 'else' type clause can be specified by using default
:
This type of functionality should be reasonably easy to understand with if-statements securely under your belt, and so if you're feeling brave - try porting the basic program we created at the start of this tutorial to use if-statements. If you're not quite that brave, the cleaner and neater switch
version of the code is below:
The switch case statement is used when we have multiple options and we need to perform a different task for each option.
C – Switch Case Statement
Before we see how a switch case statement works in a C program, let’s checkout the syntax of it.
Flow Diagram of Switch Case
Example of Switch Case in C
Let’s take a simple example to understand the working of a switch case statement in C program.
Output:
Explanation: In switch I gave an expression, you can give variable also. I gave num+2, where num value is 2 and after addition the expression resulted 4. Since there is no case defined with value 4 the default case is executed.
Twist in a story – Introducing Break statement
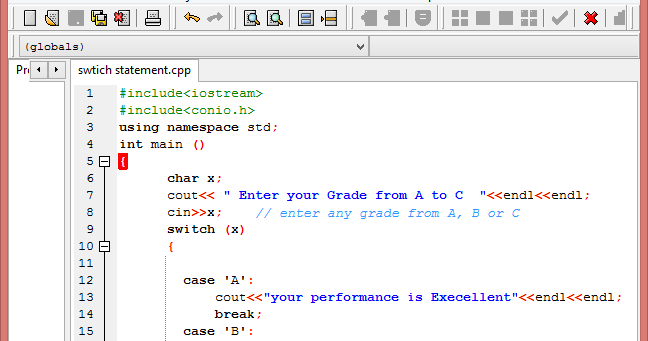
Before we discuss more about break statement, guess the output of this C program.
Output:
I passed a variable to switch, the value of the variable is 2 so the control jumped to the case 2, However there are no such statements in the above program which could break the flow after the execution of case 2. That’s the reason after case 2, all the subsequent cases and default statements got executed.
How to avoid this situation?
We can use break statement to break the flow of control after every case block.
Break statement in Switch Case
Break statements are useful when you want your program-flow to come out of the switch body. Whenever a break statement is encountered in the switch body, the control comes out of the switch case statement.
Switch Case Statement C
Example of Switch Case with break
I’m taking the same above that we have seen above but this time we are using break.
Output:
Why didn’t I use break statement after default?
The control would itself come out of the switch after default so I didn’t use it, however if you want to use the break after default then you can use it, there is no harm in doing that.
Few Important points regarding Switch Case
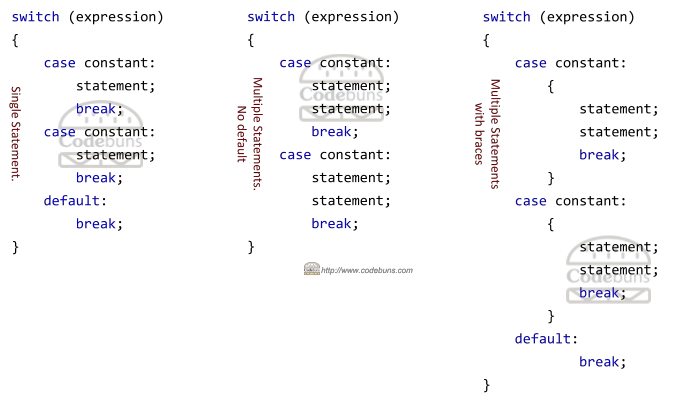
1) Case doesn’t always need to have order 1, 2, 3 and so on. They can have any integer value after case keyword. Also, case doesn’t need to be in an ascending order always, you can specify them in any order as per the need of the program.
2) You can also use characters in switch case. for example –
Dev C++ Switch Statement Examples
Output:
3) The expression provided in the switch should result in a constant value otherwise it would not be valid.
For example:
Valid expressions for switch –
C++ Switch Statement Syntax
Invalid switch expressions –
4) Nesting of switch statements are allowed, which means you can have switch statements inside another switch. However nested switch statements should be avoided as it makes program more complex and less readable.
5) Duplicate case values are not allowed. For example, the following program is incorrect:
This program is wrong because we have two case ‘A’ here which is wrong as we cannot have duplicate case values.
Switch Statement In Dev C Example Pdf
6) The default statement is optional, if you don’t have a default in the program, it would run just fine without any issues. However it is a good practice to have a default statement so that the default executes if no case is matched. This is especially useful when we are taking input from user for the case choices, since user can sometime enter wrong value, we can remind the user with a proper error message that we can set in the default statement.